If
statements are control flow statements which helps us to run a
particular code only when a certain condition is satisfied. For example,
you want to print a message on the screen only when a condition is true
then you can use if statement to accomplish this in programming.
Syntax
if condition:
block of code

Python programming language provides following types of loops to handle looping requirements. Python provides three ways for executing the loops. While all the ways provide similar basic functionality, they differ in their syntax and condition checking time.
Python has two primitive loop commands:
The Range() Function

for i in range(-10, -100, -30):
print(i)
for letter in 'python-is-Langauage':
if letter == 'p' or letter == 'o':
continue
print('Current Letter :', letter)

Break Statement: It returns the control to the beginning of the loop.
# break the loop as soon it sees 'o' or 'p'
for letter in 'python-is-Langauage':
if letter == 'o' or letter == 'P':
break
print('Current Letter :', letter)
Print i as long is less than 6
Syntax
if condition:
block of code
Python programming language provides following types of loops to handle looping requirements. Python provides three ways for executing the loops. While all the ways provide similar basic functionality, they differ in their syntax and condition checking time.
Python has two primitive loop commands:
- while loops
- for loops
The for loop in Python is used to iterate over a sequence (list,
tuple, string) or other iterable objects. Iterating over a sequence is
called traversal.In Python, there is no C style for loop, i.e., for (i=0; i<n; i++)
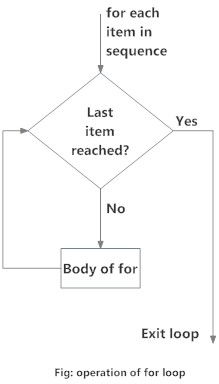
Syntax of for Loop
for val in sequence:
Body of for
fruits = ["apple", "banana", "cherry"]
for x in fruits:
print(x)
for x in fruits:
print(x)
Program to find the sum of all numbers stored in a list
numbers = [6, 5, 3, 8, 4, 2, 5, 4, 11]
sum = 0
for val in numbers:
sum = sum+val
print("The sum is", sum)
The Range() Function
If
you do need to iterate over a sequence of numbers, the built-in
function range() comes in handy. It generates arithmetic progressions:
for i in range(10):
print(i)
The given end point is never part of the generated sequence; range(10) generates 10 values, the legal indices for items of a sequence of length 10. It is possible to let the range start at another number, or to specify a different increment (even negative; sometimes this is called the ‘step’):
for i in range(5, 10):
print(i)

for i in range(0, 10, 3):
print(i)
for i in range(10):
print(i)
The given end point is never part of the generated sequence; range(10) generates 10 values, the legal indices for items of a sequence of length 10. It is possible to let the range start at another number, or to specify a different increment (even negative; sometimes this is called the ‘step’):
for i in range(5, 10):
print(i)
for i in range(0, 10, 3):
print(i)
for i in range(-10, -100, -30):
print(i)
To iterate over the indices of a sequence, you can combine range() and len() as follows:
a = ['Mary', 'had', 'a', 'little', 'lamb']
for i in range(len(a)):
print(i, a[i])
Loop Control Statements
Loop control statements change execution from its normal sequence. When
execution leaves a scope, all automatic objects that were created in
that scope are destroyed. Python supports the following control
statements.
Continue Statement: It returns the control to the beginning of the loop.
# Prints all letters except 'p' and 'o' for letter in 'python-is-Langauage':
if letter == 'p' or letter == 'o':
continue
print('Current Letter :', letter)
Break Statement: It returns the control to the beginning of the loop.
# break the loop as soon it sees 'o' or 'p'
for letter in 'python-is-Langauage':
if letter == 'o' or letter == 'P':
break
print('Current Letter :', letter)
The while loop in Python is used to iterate over a block of code as
long as the test expression (condition) is true. We generally use this
loop when we don't know the number of times to iterate beforehand.
Syntax ,.
while test expression:
body of while
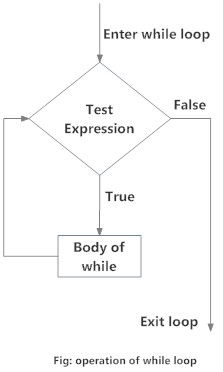
Print i as long is less than 6
i = 1
while i < 6:
print(i)
i +=1
The break Statement
i = 1
while i < 6:
print(i)
if ( i == 2):
break
i +=1
The else Statement
With the else statement we can run a block of code once when the condition no longer is true:
Print a message once the condition is false:
i = 1
while i < 6:
print(i)
i += 1
else:
print("i is no longer less than 6")
With the else statement we can run a block of code once when the condition no longer is true:
Print a message once the condition is false:
i = 1
while i < 6:
print(i)
i += 1
else:
print("i is no longer less than 6")
No comments:
Post a Comment